Crowd Fund Smart Contract Walkthrough
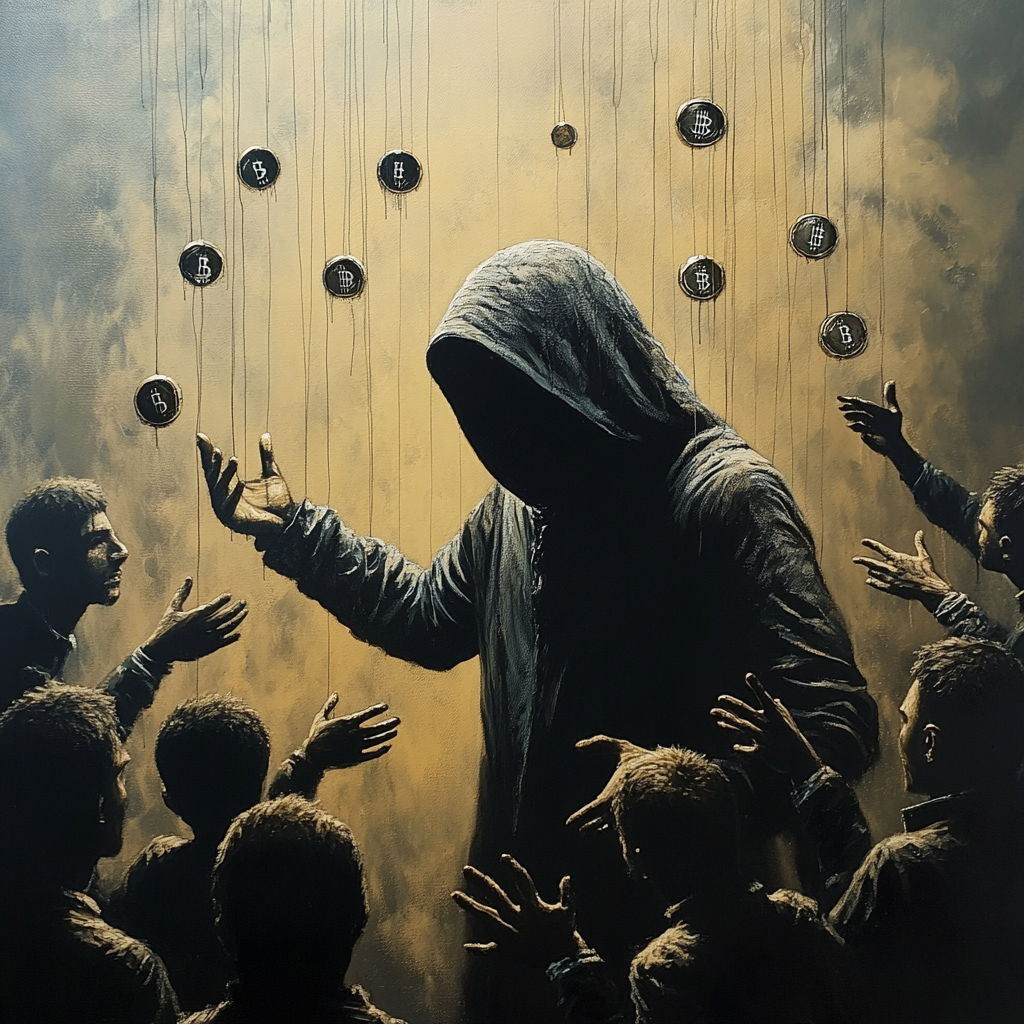
In this tutorial, we will walk through a Crowd Funding Solidity smart contract similar to applications like Kickstarter.
The code for this contract can be found at this Github Repository
Here is the functionality:
- User can create a campaign.
- Users can pledge, transferring an ERC20 token to the campaign.
- After the campaign ends, the campaign creator can claim the funds if the the total amount pledged has surpassed the campaign goal.
- Otherwise, the campaign did not reach its goal and users can withdraw their pledge.
Planning
If you continue reading my tutorials, you will notice that we spend some time mapping out the state and functionality of our contract prior to implementation.
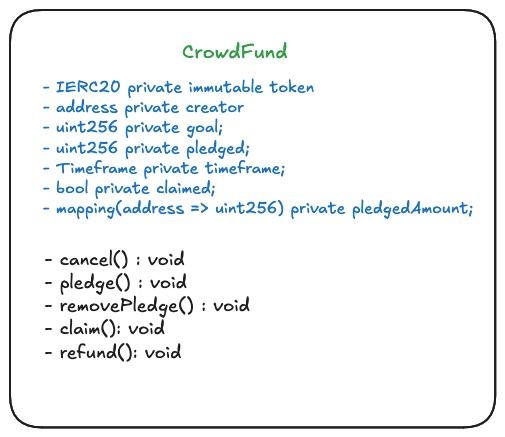
Let's walk through the the storage variables of our contract.
- token: The token fulfils the IERC20 interface. This essentially means that the token used to pledge must be an ERC20 token like USDC.
- creator: This address is the one who creates the smart contract instance.
- goal: The goal is a uint256 representing the amount of ERC20 tokens needed to be pledged in order to be considered a success.
- pledged: A uint256 representing the amount of ERC20 tokens that have been pledged to the campaign.
- timeframe: The timeframe is of type Timeframe which is a custom struct that we will define. It will store the start and end time of the campaign.
- claimed: A boolean representing if the creator has claimed the funds or not.
- pledgedAmount: A mapping of addresses to uint256 that stores the amount of tokens currently pledged by users.
Now we have some methods to discuss:
- cancel(): The contract owner may cancel the crowdfund BEFORE it has started.
- pledge(): Users can pledge a specified ERC20 token to the campaign.
- removePledge(): Users can remove their pledge while the campaign is active.
- claim(): The campaign creator can receive the pledged tokens at the end of the campaign if it was successful.
- refund(): Users can receive a refund of their pledge amount if the the campaign has ended but the goal was not achieved.
Implementation
As always, first thing we will do is declare our Licence at the top as well as our solidity compiler version. We will be using ^0.8.26.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
If you would like some information about what the pragma declaration does, see What is pragma and why it matters.
Constructor
Our constructor will take the following arguments from the contract creator.
- creator: The address of the creator will be passed into constructor. We opt in for this approach instead of simply assigning the
msg.sender
because we will create a CrowdFundFactory that will create more instances of CrowdFunds. If we did not make this change, the CrowdFundFactory would be thecreator
every time which is not something we want. - token: This is the address of the ERC20 token that will be accepted by the contract for pledges. This can be any specified ERC20 token like USDC or USDT.
- goal: The goal is of type uint256 and is the target amount of funds pledged in order for the crowdfund to be considered a success.
- timeframe: The timeframe is a custom struct that specifies the start(uint256) and end(uint256) times of the crowdfund. Since these configuration options are tightly related, we group them into a single
Timeframe
struct to reduce the number of constructor arguments and for general cleanliness.
cancel()
The cancel method sets the _cancelled
state to true. This will be checked in many other methods to avoid making changes to a cancelled fund. Before we cancel any crowd funds, we must check some things:
- Only the creator can cancel the crowdfund.
- The crowdfund has not already been cancelled.
- The crowdfund has not started already.
These checks can be done with modifiers. Lets create them now.
modifier _onlyCreator() {
if (msg.sender != _creator) {
revert("only the creator can call this function");
}
_;
}
modifier _notCancelled() {
if (_cancelled) {
revert("campaign has been cancelled");
}
_;
}
modifier _notStarted() {
if (block.timestamp >= _timeframe.start) {
revert("campaign has started");
}
_;
}
Now a closer look at each one:
- The
_onlyCreator()
modifier does a simple check: If themsg.sender
is not the_creator
, revert! - The
_notCancelled()
modifier simply checks the current state of the_cancelled
state. If the_cancelled
state is set to true, the transaction will revert. - The
_notStarted()
modifier does a check to ensure that the current time (block.timestamp
) is not greater than or equal to the start time that was set in the instructor (_timeframe.start
).
Note that in each of these the _;
specifier is at the end of the modifiers. That means that the logic should run at the beginning of the method that uses it.
Then all thats left is the logic of the method itself which is to set _cancelled
to true.
function cancel() external _onlyCreator _notCancelled _notStarted {
_cancelled = true;
}
pledge(uint256 _amount)
This method is called by anyone who wishes to pledge funds to the CrowdFund campaign.
First though we must ask if there are any cases where we DO NOT want somebody to be able to pledge.
- The campaign can not be cancelled.
- The campaign must be started.
- The campaign must have NOT ended yet.
We have a modifier _notCancelled
that we can use for case number one. Lets add the others.
modifier _started() {
if (block.timestamp < _timeframe.start) {
revert("campaign has not started");
}
_;
}
modifier _notEnded() {
if (block.timestamp >= _timeframe.end) {
revert("campaign has ended");
}
_;
}
_started()
checks if the current timestamp (block.timestamp
) is less than the start time of the campaign (_timeframe.start
). If true, the campaign has not started yet._notEnded()
checks if the current time (block.timestamp
) is greater than the end time of the campaign (_timeframe.end
). If true, the campaign has already ended.
Here is the full pledge() method:
function pledge(uint256 _amount) external _notCancelled _started _notEnded {
_token.transferFrom(msg.sender, address(this), _amount);
_pledgedAmount[msg.sender] += _amount;
_pledged += _amount;
}
Let's break it down:
- Transfers a specified amount (
_amount
) of ERC20 tokens from the message sender (msg.sender
) to the CrowdFund contract instance (address(this)
. - The state variable we have mapping pledged amounts to addresses (
_pledgedAmount
) is updated. - The state variable we have holding the total pledged amount
_pledged
is updated.
removePledge()
This method is called when somebody who has already pledged, would like to withdraw their pledge and receive their funds back. Are there any conditions where we want to restrict this method from being called?
It turns out, they are exactly the same as the pledge()
method so lets just add the same modifiers to the removePledge()
method.
function removePledge(uint256 _amount) external _notCancelled _started _notEnded {
_pledgedAmount[msg.sender] -= _amount;
_pledged -= _amount;
_token.transfer(msg.sender, _amount);
}
Heres a recap:
- In our mapping of addresses to their pledged amounts (
_pledgedAmount
), we reduce the specified amount to unpledge (_amount
). - Decrease the specified amount (
_amount
) from the total pledged (_pledged
). - Call the token contract (
_token
) and transfer the specified amount (_amount
) from the CrowdFund Instance to the message sender (_msg.sender
).
**NOTE: When I reviewed this method, I had a bit of a panic. What if somebody calls the removePledge() method and they have nothing pledged!? The logic doesn't explicitly check this! Well, fortunately with the current version of the Solidity compiler, we are protected from overflows and underflows. When the pledgedAmount for the address is decreased from zero, an error occurs and the transaction is reverted.
claim()
This method is called by the contract creator to claim the funds that were pledged to the campaign.
We need to check some things again here:
- Only the creator can call this method.
- The campaign must NOT have been cancelled.
- The campaign must be started.
- The pledge goal must have ben reached.
- The funds must NOT have been claimed.
We already have modifiers for the first three, so let's just add the other two.
modifier _goalReached() {
if (_pledged < _goal) {
revert("goal has not been reached");
}
_;
}
modifier _notClaimed() {
if (_claimed) {
revert("campaign has been claimed");
}
_;
}
Heres the full method:
function claim() external
_onlyCreator
_notCancelled
_started
_goalReached
_notClaimed {
_claimed = true;
_token.transfer(_creator, _pledged);
}
It's quite straightforward:
- We change our state variable
_claimed
to true. - We call the token contract (
_token
) in order to transfer the total pledged amount (_pledged
) to the contract creator (_creator
).
refund()
This method is called by someone who has pledged funds and wishes to get a refund after the campaign has ended and the campaign goal has not been met. As usual, lets think of the conditions that must be met in order for this transaction to be processed.
- The campaign must NOT be cancelled.
- The campaign must have started.
- The campaign must have ended.
- The funds must NOT have been cancelled
- The campaign goal must have NOT been reached.
We have modifiers in place for the first four that we can reuse. Let's add one more.
modifier _goalNotReached() {
if (_pledged >= _goal) {
revert("goal has been reached");
}
_;
}
And for the full method:
function refund() external _notCancelled _notClaimed _started _ended _goalNotReached {
uint256 pledged = _pledgedAmount[msg.sender];
_token.transfer(msg.sender, pledged);
_pledgedAmount[msg.sender] = 0;
}
- Obtain the pledged amount for the message sender (
_pledgedAmount[msg.sender]
). - Call the token contract (
_token
) to transfer the pledged amount to the message sender. - We reduce the pledged amount of the message send to 0.
And with that, we have our CrowdFund smart contract! If you followed along, congratulations. In the repository, there are some additions. Namely, there is a new smart contract called CrowdFundFactory.sol that creates new instances of the CrowdFund contract. In another post I will explain why this was added but for now, take a break and grab a cup of coffee. See you on the next one.
The full contract:
import {IERC20} from "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import {Timeframe} from "./TimeFrame.sol";
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
contract CrowdFund {
IERC20 private immutable _token;
address private immutable _creator;
uint256 private immutable _goal;
uint256 private _pledged;
Timeframe private _timeframe;
bool private _claimed;
bool private _cancelled;
mapping(address => uint256) private _pledgedAmount;
constructor(address c_creator, address c_token, uint256 c_goal, Timeframe memory c_timeframe) {
if (c_creator == address(0)) {
revert("creator must be a valid address");
}
if (c_timeframe.start > c_timeframe.end) {
revert("start must be before end");
}
if (c_timeframe.start <= block.timestamp) {
revert("start must be in the future");
}
if (c_timeframe.end > block.timestamp + 90 days) {
revert("end must be within 90 days");
}
if (c_goal <= 0) {
revert("goal must be greater than 0");
}
if (c_token == address(0)) {
revert("token must be a valid address");
}
_token = IERC20(c_token);
_goal = c_goal;
_timeframe = c_timeframe;
_creator = c_creator;
}
function cancel() external _onlyCreator _notCancelled _notStarted {
_cancelled = true;
}
function pledge(uint256 _amount) external _notCancelled _started _notEnded {
_token.transferFrom(msg.sender, address(this), _amount);
_pledgedAmount[msg.sender] += _amount;
_pledged += _amount;
}
function removePledge(uint256 _amount) external _notCancelled _started _notEnded {
_pledgedAmount[msg.sender] -= _amount;
_pledged -= _amount;
_token.transfer(msg.sender, _amount);
}
function claim() external
_onlyCreator
_notCancelled
_started
_goalReached
_notClaimed {
_claimed = true;
_token.transfer(_creator, _pledged);
}
function refund() external _notCancelled _notClaimed _started _ended _goalNotReached {
uint256 pledged = _pledgedAmount[msg.sender];
_token.transfer(msg.sender, pledged);
_pledgedAmount[msg.sender] = 0;
}
function cancelled() public view returns (bool) {
return _cancelled;
}
function pledged() public view returns (uint256) {
return _pledged;
}
function creator() public view returns (address) {
return _creator;
}
function pledgedAmount(address _address) public view returns (uint256) {
return _pledgedAmount[_address];
}
function endTime() public view returns (uint256) {
return _timeframe.end;
}
function startTime() public view returns (uint256) {
return _timeframe.start;
}
function token() public view returns (address) {
return address(_token);
}
function goal() public view returns (uint256) {
return _goal;
}
modifier _onlyCreator() {
if (msg.sender != _creator) {
revert("only the creator can call this function");
}
_;
}
modifier _notCancelled() {
if (_cancelled) {
revert("campaign has been cancelled");
}
_;
}
modifier _started() {
if (block.timestamp < _timeframe.start) {
revert("campaign has not started");
}
_;
}
modifier _notStarted() {
if (block.timestamp >= _timeframe.start) {
revert("campaign has started");
}
_;
}
modifier _notEnded() {
if (block.timestamp >= _timeframe.end) {
revert("campaign has ended");
}
_;
}
modifier _ended() {
if (block.timestamp < _timeframe.end) {
revert("campaign has not ended");
}
_;
}
modifier _goalReached() {
if (_pledged < _goal) {
revert("goal has not been reached");
}
_;
}
modifier _goalNotReached() {
if (_pledged >= _goal) {
revert("goal has been reached");
}
_;
}
modifier _notClaimed() {
if (_claimed) {
revert("campaign has been claimed");
}
_;
}
}