English Auction Smart Contract Walkthrough
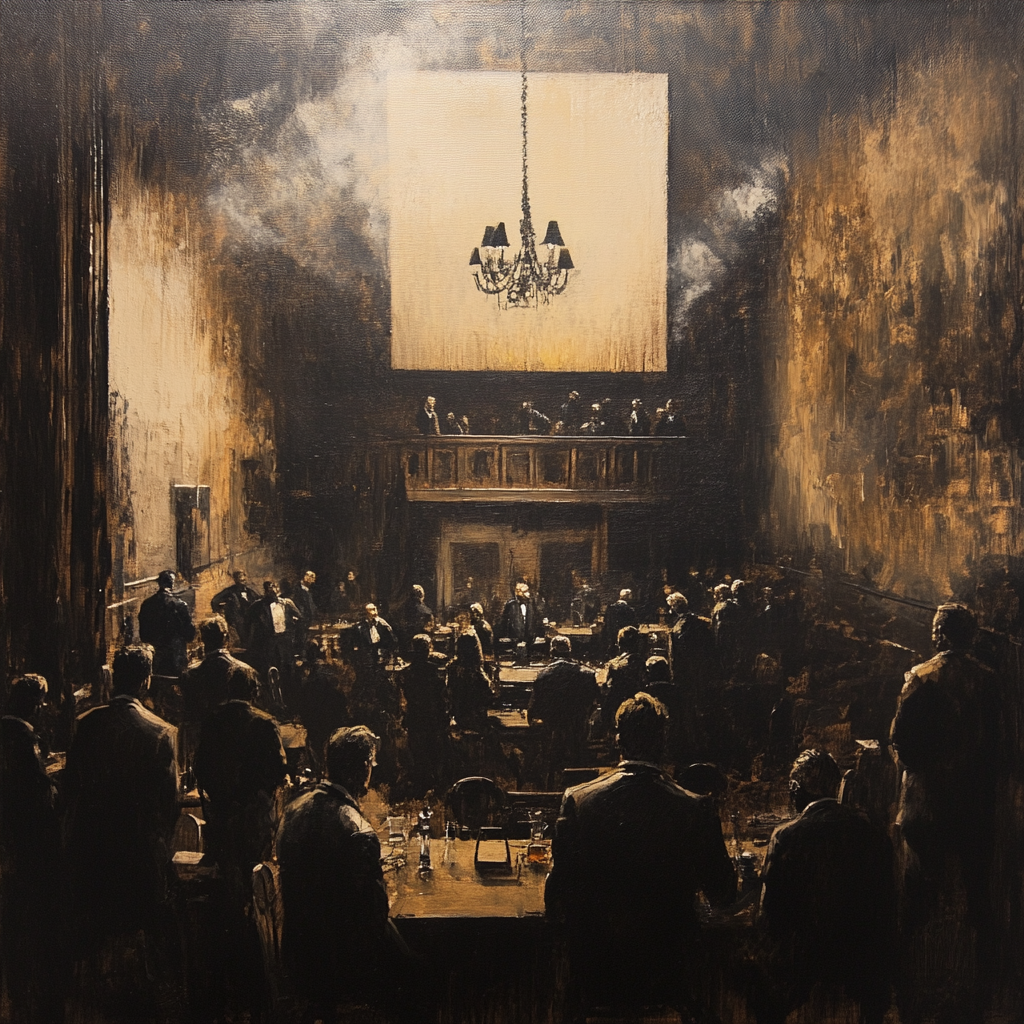
This smart contract is an implementation of an English Auction. It does the following:
Auction
- Seller of an NFT deploys the contract
- The Auction lasts for 7 days.
- Participants can bid by depositing ETH greater than the current highest bidder.
- All bidders can withdraw their bid if it is not the current highest bid.
After the auction
- The highest bidder becomes the new owner of the NFT
- The seller receives the highest bid of ETH.
The full code including tests can be found at this Github Repository.
We will be learning about some new topics here such as interfaces, ERC721 tokens, mappings and block timestamps. So grab a cup of coffee and let's write some code!
Planning
Before we begin writing our contract, we need to think about who our contract is. Notice that I say who instead of what. Out contract, once deployed will be on its own in the world and will behave as an independent organism that we need to trust to do its job.
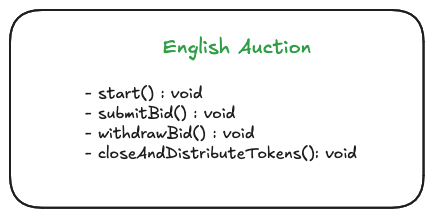
This image describes the public methods that our English Auction contract will expose. It does not include internal state and methods.
- start(): Sets the endTime of the auction and opens the auction for bids.
- submitBid(): Users can submit a bid to the Auction. The highestBidder state is updated as well as the highestBid state.
- withdrawBid(): A bidder who is not currently the highest bidder removes their ETH from the auction contract.
- closeAndDistributeTokens(): The auction is closed to additional bids. The highest bidder is sent the NFT and the bid is sent to the seller. If no bids exist, the NFT is sent back to the seller.
Implementation
As always, first thing we will do is declare our Licence at the top as well as our solidity compiler version. We will be using ^0.8.26.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
If you would like some information about what the pragma declaration does, see What is pragma and why it matters.
State, Constructor
The state and constructor will consist of various private state variables that make the Auction instance unique. We will go over the following code:
contract EnglishAuction {
IERC721 private _nft;
uint256 private _nftId;
address payable private _seller;
uint256 private _endAt;
bool private _started;
bool private _closed;
address private _highestBidder;
uint256 private _highestBid;
mapping(address => uint256) private _bids;
constructor(address _nftContract, uint256 _nftIdentifier, uint256 startingBid) {
_nft = IERC721(_nftContract);
_nftId = _nftIdentifier;
_seller = payable(msg.sender);
_highestBid = startingBid;
}
}
- _nft:
_nft
is of type IERC721. What is that?? Typically, if a data type is prefixed with "I" then it is an interface. Let's talk about interfaces briefly.
A contract is a living organism in the blockchain universe (in our case, Ethereum). It communicates with organisms and assists them in performing their work. Other organisms can also help our contract do it's work. This make's it a very social, tight environment.
Contracts are coupled to each other due to their inherent need for each other. Everything is fine and dandy when we know exactly what each contract has to do and what services he has to provide to other contracts. However, as more and more contracts are created with different variations, tight coupling of contracts becomes a serious issue. This makes building contracts on Ethereum inflexible so we have to do our best to decouple the contracts. This means that we should be able to modify one contract without modifying the others that interact with it. The best way to achieve this is via interfaces. In our EnglishAuction contract, we depend on an ERC721 nft being assigned to to. An ERC721 contract is one that fulfils the following contract:
It should have at least the following methods:
interface IERC721 {
function safeTransferFrom(address from, address to, uint256 tokenId)
external;
function transferFrom(address, address, uint256) external;
}
Lets import that in our solidity file above our contract so that the contract is aware of this interface. The file should now look like this:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.13;
interface IERC721 {
function safeTransferFrom(address from, address to, uint256 tokenId)
external;
function transferFrom(address, address, uint256) external;
}
contract EnglishAuction {
IERC721 private _nft;
uint256 private _nftId;
address payable private _seller;
uint256 private _endTime;
bool private _started;
bool private _closed;
address private _highestBidder;
uint256 private _highestBid;
mapping(address => uint256) private _bids;
constructor(address _nftContract, uint256 _nftIdentifier, uint256 startingBid) {
_nft = IERC721(_nftContract);
_nftId = _nftIdentifier;
_seller = payable(msg.sender);
_highestBid = startingBid;
}
}
On to the next state variables:
- _nftId: The nftId is of type uint256. It is different from the
nft
variable which is a reference to the nft contract itself. The nft contract represents an nft collection. Perhaps it is the CryptoPunks contract. ThenftId
represents a specific nft in the collect. For example, if $nftId = 42$, it refers to CryptoPunk # 42. - _seller: A payable address that represent the contract or address auctioning the nft.
- _endTime: A uint256 representing the block timestamp that the auction ends.
- _started: A boolean that represents if the auction is open for bids.
- **_closed: A boolean that represents if the auction has closed and no longer accepts bids.
- _highestBidder: The address of the person who has placed the highest bid at any given point in time.
- _highestBid: A unit256 representing the hightest bid at any given point in time.
- _bids: A map of current bidder addresses and their current bids.
In the constructor, we accept things necessary to kick start the auction. We initialize the nft contract, the nftId and the starting bid which is the minimum bid offer that the seller is willing to accept. This prevents someone from winning the auction with an extremely low bid.
Methods
Now with the setup out of the way, we can begin adding functionality to the contract.
start()
We will set the _started
variable to true and we will set the _endTime
to 7 days from now - block.timestamp + 7 days
. We will also send the nft from the seller to the address.
However, we don't want just anybody to be able to start this auction right? No, that would be bad, after all this is the sellers auction. We also do not want the seller to be able to call this method in 5 days and reset the endTime!!
We will create modifiers for this. Modifiers are checks done before or after a contracts method is executed. In our case, we have two checks:
- Only the seller can start the auction.
- The seller can only start the auction if
_started
is set to false.
Lets add these modifiers to our code:
modifier _onlySeller() {
require(msg.sender == _seller, "not seller");
_;
}
modifier _auctionNotStarted() {
require(!_started, "started");
_;
}
The _;
is a special character that represents the rest of the method logic. So placing it before the require()
would mean that we want the modifier to run at the end of the method. However, we want these checks to be done at the beginning of the method so that if the assertion fails, we save gas by avoiding the rest of the computation.
Now, our start()
function looks like this:
function start() external _onlySeller _auctionNotStarted {
_started = true;
_endAt = block.timestamp + 7 days;
}
Pretty simple to read right?! And it is secure thanks to our nifty modifiers.
submitBid()
Now lets create our submitBid()
method. Note that it must be payable
as the sender will attach ETH to the transaction. It will do the following:
- If there is already a highest bidder, (i.e. the highestBidder address is not a 0 address), we want to add the current highest bidder and their bid amount to our
_bids
map. This is important, because in order for somebody to withdraw their bid, it must be in the_bids
map. - Set
_highestBidder
to the message sender. - Set
_highestBid
to the msg.value.
But WAIT! What aren't there some things we should check before anyone can just go adding bids!?? Well yes, in fact we should check the following:
- The auction has actually STARTED (
_started = true
) - The auction endTime has not passed (
block.timestamp < _endTime
) - The person creating the bid is submitting a value that is GREATER than the current highest bid (
msg.value > _highestBid
)
Let's create some more modifiers:
modifier _auctionStarted() {
require(_started, "not started");
_;
}
modifier _auctionEndTimeNotReached() {
require(block.timestamp < _endAt, "ended");
_;
}
modifier _bidLargerThanHighestBid() {
require(msg.value > _highestBid, "bid not larger than highest bid");
_;
}
Now let's put them all together:
function submitBid() external payable _auctionStarted _auctionEndTimeNotReached _bidLargerThanHighestBid {
_highestBidder = msg.sender;
_highestBid = msg.value;
}
Once again, nice and easier to understand what is happening.
withdrawBid()
Now we need to give bidders the ability to withdraw their bid. It does the following:
- Grabs the current bid of the sender (
_bids[msg.sender]
); - Sets the senders current bid to 0 (
_bids[msg.sender] = 0
); - Transfers the current bid back to the sender (`payable(msg.sender).transfer(currentBid));
It will look like this:
function withdrawBid() external {
uint256 currentBid = _bids[msg.sender];
_bids[msg.sender] = 0;
payable(msg.sender).transfer(currentBid);
}
close()
Finally, we want to have the ability to close the auction and send the NFT and funds to the correct address (either the highest bidder if one exists, or back to the seller).
- Close the auction (
_closed = true
) - If there is a highest bidder
- Transfer the NFT to the highest bidder.
- Transfer the highest bid to the seller
- If there is no highest bidder
- Transfer the NFT back to the seller.
Are we missing anything?? Some more safety checks maybe? Here's what we need to check.
- The auction has started
- The current timestamp is greater than the
_endTime
set when the auction started. - The auction is not already closed.
Lets use modifiers to separate the logic and keep things clean.
We already have an _auctionStarted
modifier so lets reuse that.
modifier _auctionEndTimeReached() {
require(block.timestamp >= _endAt, "end time not reached");
_;
}
modifier _auctionNotClosed() {
require(!_closed, "closed");
_;
}
Our close() method now looks like this:
function closeAndDistributeTokens() external _auctionStarted _auctionNotClosed _auctionEndTimeReached {
_closed = true;
if (_highestBidder != address(0)) {
_nft.safeTransferFrom(address(this), _highestBidder, _nftId);
_seller.transfer(_highestBid);
} else {
_nft.safeTransferFrom(address(this), _seller, _nftId);
}
}
Getters
Now, since all of our state variables are private, we want to expose the information we want to other contracts.
function nft() external view returns (address) {
return address(_nft);
}
function nftId() external view returns (uint256) {
return _nftId;
}
function highestBid() external view returns (uint256) {
return _highestBid;
}
function highestBidder() external view returns (address) {
return _highestBidder;
}
function endAt() external view returns (uint256) {
return _endAt;
}
function started() external view returns (bool) {
return _started;
}
function closed() external view returns (bool) {
return _closed;
}
function seller() external view returns (address) {
return _seller;
}
function bidsByBidder(address bidder) external view returns (uint256) {
return _bids[bidder];
}
And everything together now!
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.13;
interface IERC721 {
function safeTransferFrom(address from, address to, uint256 tokenId)
external;
function transferFrom(address, address, uint256) external;
}
contract EnglishAuction {
IERC721 private _nft;
uint256 private _nftId;
address payable private _seller;
uint256 private _endAt;
bool private _started;
bool private _closed;
address private _highestBidder;
uint256 private _highestBid;
mapping(address => uint256) private _bids;
constructor(address _nftContract, uint256 _nftIdentifier, uint256 startingBid) {
_nft = IERC721(_nftContract);
_nftId = _nftIdentifier;
_seller = payable(msg.sender);
_highestBid = startingBid;
}
function start() external _onlySeller _auctionNotStarted {
_started = true;
_endAt = block.timestamp + 7 days;
_nft.transferFrom(msg.sender, address(this), _nftId);
}
function submitBid() external payable _auctionStarted _auctionEndTimeNotReached _bidLargerThanHighestBid {
_highestBidder = msg.sender;
_highestBid = msg.value;
}
function withdrawBid() external {
uint256 currentBid = _bids[msg.sender];
_bids[msg.sender] = 0;
payable(msg.sender).transfer(currentBid);
}
function closeAndDistributeTokens() external _auctionStarted _auctionNotClosed _auctionEndTimeReached {
_closed = true;
if (_highestBidder != address(0)) {
_nft.safeTransferFrom(address(this), _highestBidder, _nftId);
_seller.transfer(_highestBid);
} else {
_nft.safeTransferFrom(address(this), _seller, _nftId);
}
}
function nft() external view returns (address) {
return address(_nft);
}
function nftId() external view returns (uint256) {
return _nftId;
}
function highestBid() external view returns (uint256) {
return _highestBid;
}
function highestBidder() external view returns (address) {
return _highestBidder;
}
function endAt() external view returns (uint256) {
return _endAt;
}
function started() external view returns (bool) {
return _started;
}
function closed() external view returns (bool) {
return _closed;
}
function seller() external view returns (address) {
return _seller;
}
function bidsByBidder(address bidder) external view returns (uint256) {
return _bids[bidder];
}
modifier _onlySeller() {
require(msg.sender == _seller, "not seller");
_;
}
modifier _auctionNotStarted() {
require(!_started, "started");
_;
}
modifier _auctionStarted() {
require(_started, "not started");
_;
}
modifier _auctionEndTimeNotReached() {
require(block.timestamp < _endAt, "ended");
_;
}
modifier _bidLargerThanHighestBid() {
require(msg.value > _highestBid, "bid not larger than highest bid");
_;
}
modifier _auctionNotClosed() {
require(!_closed, "closed");
_;
}
modifier _auctionEndTimeReached() {
require(block.timestamp >= _endAt, "end time not reached");
_;
}
}
Congratulations! This English Auction contract covers a lot of different topics fundamental to your Smart Contract Engineer journey.
NOTE: In the Github repository you will see some changes made to the IERC721 interface so that tests will work properly.