Ether Wallet Smart Contract Walkthrough
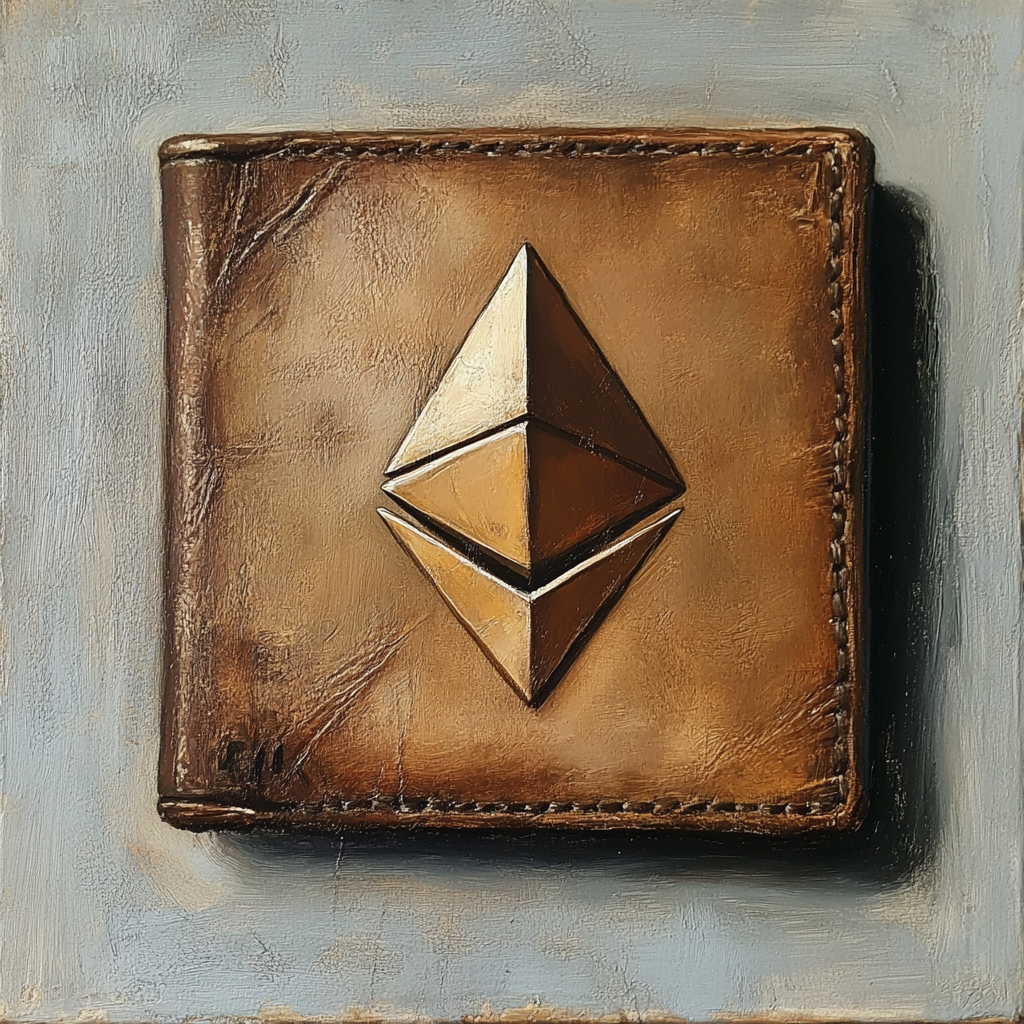
This smart contract is an implementation of a basic wallet. It does the following:
- Anyone can send ETH.
- Only the owner can withdraw.
The full code including tests can be found at this Github Repository.
It makes sense for us to map out what our contract will look like.
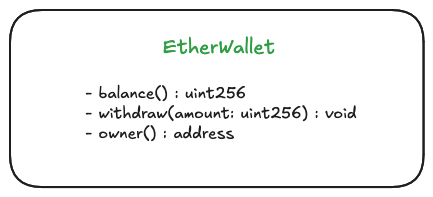
When defining our contract, we can think of it in a similar way to defining a Class in other languages. We must treat our smart contracts as living beings and treat them with respect. We have planned the following methods for our contract.
balance(): uint256,
withdraw(amount: uint256): void
owner(): address
Basically, we want our wallet instances to be able to retrieve the ETH balance in the wallet. We want the owner of the wallet to have the ability to withdraw ETH from the wallet. We also want anybody to be able to retrieve the owners address.
The first thing we will do is declare our Licence at the top as well as our solidity compiler version. We will be using ^0.8.26.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
If you would like some information about what the pragma declaration does, see What is pragma and why it matters.
State and Constructor
We can now begin the creation of our contract by creating the state and the constructor. The state is responsible for storing contract data that makes the contract instance unique. In our case, what separates one wallet from another wallet is, for simplicity, the owner of the contract. The owner of the contract is the only one who can withdraw ETH and as a result, owns the wallet. The owner will be set at the creation of the EtherWallet contract via the constructor. Here is the code so far:
contract EtherWallet {
address payable private _owner;
constructor() {
_owner = payable(msg.sender);
}
}
There are a couple of things we haven't discussed so far so lets go over them now.
In Ethereum, every smart contract and wallet (Externally Owned Account) has an address which as treated as its own type: address. When being used inside of a smart contract, an address can either be payable or not payable. If an address is payable, then the smart contract using it will treat it as an address that can send and receive ETH. It's worth mentioning that payable
is an ETH specific keyword. So it is unrelated to whether or not the address can send or receive other tokens such as ERC-20 tokens.
Next, we also call the _owner
private. The underscore is a convention in solidity that signifies that a variable is private. This means that other contracts CAN NOT access it. The private
keyword only restricts access within the smart contract itself. In other words, it doesn't encrypt or hide the data on-chain. In basically all of the smart contracts I create, I will be making my storage variables private. This is for a couple of reasons.
- We want to keep internal details hidden from other contracts.
- We want to treat our contracts with respect and we do not wish to treat it as a glorified data structure. We do not want to make assumptions about it's internals work or how it prepares data for us.
Next, we have our constructor where we set the owner
storage variable. We now know what payable
does, so we can assume that msg.sender
is an address but and by the name we can assume it is the sender of the transaction, which is the correct assumption. Where does it come from though?
msg.sender
is like the caller ID in a phone call. It stores the account or contract that called the function and it comes from the global msg object, which Solidity provides for transaction details.
withdraw()
Now we can implement the withdraw
method. The withdraw method will accept an amount as a uint256 and will not return anything. We also want to be sure that only the _owner
can withdraw funds. Lets add this to the contract
function withdraw(uint256 _amount) external {
require(msg.sender == _owner, "caller is not owner");
_owner.transfer(_amount);
}
The external keyword defines the visibility of the method. A function marked as external can only be called from outside of the contract. It cannot be called internally by other methods within the same contract or by contracts that inherit it.
The first thing we do in the method is use require()
. require() is a guard clause that halts execution and reverts the transaction if its condition is false. In this case, it prevents non-owners from withdrawing ETH.
Since _owner
is of type address payable, it has the following methods available:
address.transfer(amount)
: Sends ETH to the address and reverts if the transaction fails.address.send(amount)
: Sends ETH to the address but does not revert on failure. It instead returns a boolean (true if successful, false if failed).
In our EtherWallet contract, we want the the transaction to revert if there is a failure so we use transfer
.
balance()
Next up, we will implement the balance
method. It is external so only other contracts or accounts can call it. There is a keyword we haven't discussed yet called view
. view is a keyword in Solidity used to declare a read-only function. This means that the function doesn't modify the state of the blockchain, and only reads data from the blockchain. The method returns a uint256.
function balance() external view returns (uint256) {
return address(this).balance;
}
To retrieve the balance we use address(this)
. this refers to the contract's address. It is a reference to the current instance of the contract that is executing the code. We use the address()
code to cast the address string to an address type (which is a type for Ethereum addresses). Once it is casted to an address type, we can access the .balance
property which returns the Ether balance of an address. In our case, it returns the balance of the EtherWallet contract.
A quick note, in most Solidity code you will see, the standard naming convention for a method like this is getBalance()
, instead of my preferred balance()
. I have philosophical reasons for this decision that have to do with standards regarding how we treats our contracts with respect and request what we want to receive from the contract instead of demanding the contract to do something. The contract is not a bag of data but an autonomous organism that lives on chain and we should treat it with respect. I will create another post at some point explaining further.
owner()
Finally, we will create a function that returns the owner of the address.
function owner() external view returns (address) {
return _owner;
}
If you have been following along this far, I think the code is quite clear.
Now, there is one more thing that we have to add to our contract that isn't extremely obvious. We need to add this method to the contract:
receive() external payable {}
The receive() function is a special fallback function in Solidity that allows the contract to receive ETH when it's sent without any data. (i.e. a plain ETH transfer). If a contract does not have a receive() function (or a_ fallback() function that is payable), it cannot receive plain ETH transfers (e.g., via .transfer() or .send()).
Here is the final contract code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.13;
contract EtherWallet {
address payable private _owner;
constructor() {
_owner = payable(msg.sender);
}
receive() external payable {}
function withdraw(uint256 _amount) external {
require(msg.sender == _owner, "caller is not owner");
_owner.transfer(_amount);
}
function balance() external view returns (uint256) {
return address(this).balance;
}
function owner() external view returns (address) {
return _owner;
}
}
That completes our simple Ether wallet! Despite it's simplicity, we covered quite a few topics that will serve as a backbone during our Smart Contract Engineering journey. See you on the next one!