Dutch Auction Smart Contract Walkthrough
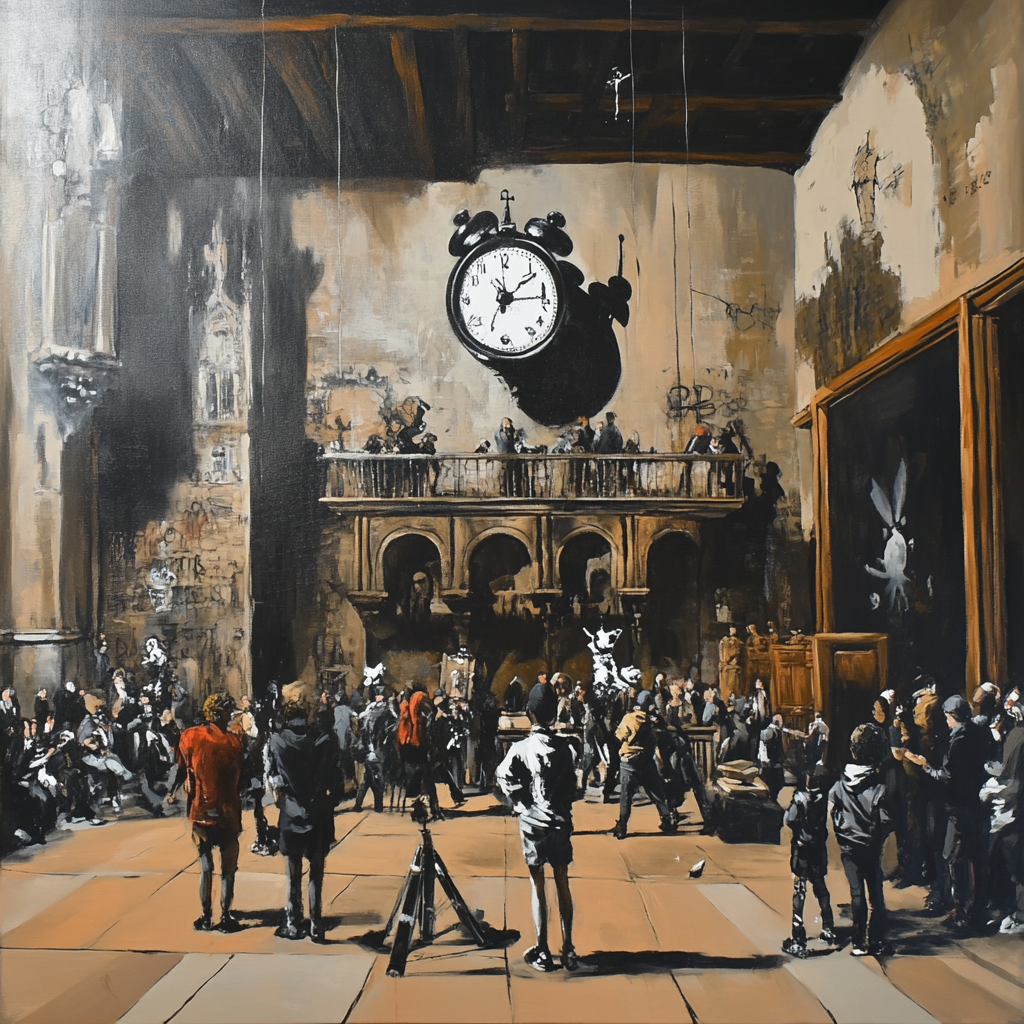
In this blog post we will be going over walking through, step by step, building a Dutch Auction in Solidity. This tutorial will resemble a recent blog post that went over how to build an English Auction smart contract. If you are interested, I suggest you check it out!
The code for this contract can be found at this Github Repository.
The Dutch Auction smart contract will do the following:
- The seller of an NFT will deploy this contract and sets a starting price for an NFT.
- The auction lasts 7 days.
- The price of the NFT decreases over time.
- A participant can buy the NFT by depositing an amount of ETH greater than the current price computed by the smart contract.
- The auction ends when a buyer buys the NFT or the auction expires.
Planning
As always we must think about our smart contract as a living organism. What is necessary for our contract to fulfil its purpose? Lets map it out.
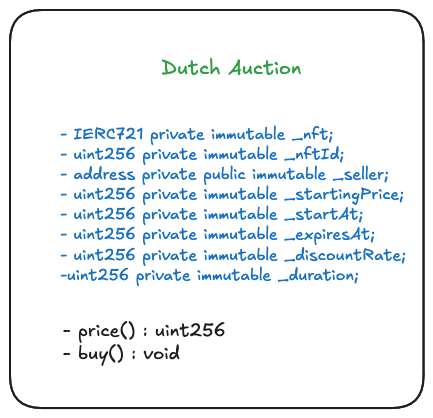
What makes any contract is it's state and its functionality. Here we have quite a few state variables as well as some methods. It is these methods that I care about the most as that is what the outside world will be interacting with. We will create some additional methods that expose the state data to others but it will be in our own control. That is why all of these state variables are private. We treat our contracts with respect and we do not simply give others the ability to look at it naked. If someone else wants something from our contract they will need to ask for it.
Lets go over our state variables.
- _nft: This is the nft contract and it is of type IERC721. This is an interface and I explain it in more detail in the English Auction Smart Contract.
- _nftId: The nftId is of type uint256. It is different from the
nft
variable which is a reference to the nft contract itself. The nft contract represents an nft collection. Perhaps it is the CryptoPunks contract. ThenftId
represents a specific nft in the collect. For example, ifnftId = 42
, it refers to CryptoPunk # 42. - _seller: A payable address that represent the contract or address auctioning the nft.
- _startingPrice: The starting price is of type uint256 is set by the seller during contract creation.
- _startTime: The start time is of type uint256 and is set during contract creation by taking the block timestamp.
- _expireTime: The expire time is of type uint256 and is set during contract creation by adding the block timestamp and the duration. NOTE: We will be hardcoding the duration as a constant in this example.
- _discountRate: The discount rate is of type uint256 and is passed into the constructor of the contract.
There is a keyword here I haven't spoken about that is the immutable keyword. This means that the variables will be stored in bytecode instead of storage. This saves on gas on every read operation. Immutable variables are great and you should make a state variable immutable any chance you get. This is not just because of the gas savings but because it prevents accidental or malicious modifications. It is clean.
Implementation
As always, first thing we will do is declare our Licence at the top as well as our solidity compiler version. We will be using ^0.8.26.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.26;
If you would like some information about what the pragma declaration does, see What is pragma and why it matters.
State and Constructor
Our constructor will take the following arguments from the contract creator.
- Starting Price
- Discount Rate
- NFT contract
- NFT Id
The constructor will then use this data to set the state variables. However the constructor must check that the starting price is greater than or equal to the discount rate * the duration. Here is an example:
_startingPrice = 7000 ETH _discountRate = 0.01 ETH DURATION = 7 days (604800 seconds)
Therefore, the minimum price that the NFT will reach is:
_minPrice = _discountRate * DURATION = 0.01 * 604800 = 6048 ETH
Since 7000 ETH >= 6048 ETH everything is GOOD! This means that the price of the NFT won't be negative by the end of the auction.
Here is our code so far.
contract DutchAuction {
uint256 private constant DURATION = 7 days;
IERC721 private immutable _nft;
uint256 private immutable _nftId;
address payable private immutable _seller;
uint256 private immutable _startingPrice;
uint256 private immutable _startAt;
uint256 private immutable _expiresAt;
uint256 private immutable _discountRate;
constructor(
uint256 c_startingPrice,
uint256 c_discountRate,
address c_nft,
uint256 c_nftId
) {
_seller = payable(msg.sender);
_startingPrice = c_startingPrice;
_startAt = block.timestamp;
_expiresAt = block.timestamp + DURATION;
_discountRate = c_discountRate;
require(
_startingPrice >= _discountRate * DURATION, "starting price < min"
);
_nft = IERC721(c_nft);
_nftId = c_nftId;
}
}
price()
Our price()
method is responsible for calculating the current price of the NFT offered by the dutch auction and returning it as a uint256. The method is public which means that it can be called internally and externally. We will need this method in the next method we create.
function price() public view returns (uint256) {
uint256 timeElapsed = block.timestamp - _startAt;
uint256 discount = _discountRate * timeElapsed;
return _startingPrice - discount;
}
Lets walk through the logic here:
- We calculation the
timeElapsed
. This is the timestamp at the time of callingprice()
minus the time when the auction started (_startAt
). - Next, we use the time elapsed to calculate the
discount
by multiplying the discount rate (_discountRate
) by thetimeElapsed
. - Finally we return the price by subtracting the discount from the starting price (
_startingPrice - discount
).
buy()
The buy
method is responsible for letting a participant purchase the NFT at the current price (we will use our price()
method) and transfer the NFT from the user to the message sender. The method will also check if the purchaser sent more ETH than required and refund the extra ETH.
Before we run this method, we need to check that the auction has not expired yet. This is a great opportunity to use a modifier to keep the method as clean as possible.
modifier _auctionNotExpired() {
require(block.timestamp < _expiresAt, "auction expired");
_;
}
Our modifier checks that the current block timestamp is less than the expiry time of the auction. This assertion fails, it will return the error message "auction expired".
We place the _; at the end of the method so because the _; is a placeholder for "the rest of the method logic";
Here is our buy()
method:
function buy() external payable _auctionNotExpired {
uint256 currentPrice = price();
require(msg.value >= currentPrice, "ETH < price");
_nft.transferFrom(_seller, msg.sender, _nftId);
uint256 refund = msg.value - currentPrice;
if (refund > 0) {
payable(msg.sender).transfer(refund);
}
}
Again, lets simply walkthrough the logic:
- The
_auctionNotExpired
modifier checks that the auction is not yet expired. - It calls
price()
to obtain the current price of the NFT factoring the discount. - It calls the nft contract to transfer the nft from the seller to the buyer(
msg.sender
). - It calculates any extra amount that the buyer (
msg.sender
) sent in the traction that exceeds the current price of the nft. - If there is an amount to refund (
refund > 0
), transfer the refund amount to the message sender.(payable(msg.sender).transfer(refund)
)
Here is the final code including the IERC721 interface that is needed for our tests.
// SPDX-License-Identifier: UNLICENSED
pragma solidity ^0.8.13;
interface IERC721 {
function safeTransferFrom(address from, address to, uint256 tokenId) external;
function transferFrom(address, address, uint256) external;
function mint(address to, uint256 tokenId) external;
function approve(address to, uint256 tokenId) external;
function ownerOf(uint256 tokenId) external view returns (address);
}
contract DutchAuction {
uint256 private constant DURATION = 7 days;
IERC721 private immutable _nft;
uint256 private immutable _nftId;
address payable private immutable _seller;
uint256 private immutable _startingPrice;
uint256 private immutable _startAt;
uint256 private immutable _expiresAt;
uint256 private immutable _discountRate;
constructor(
uint256 c_startingPrice,
uint256 c_discountRate,
address c_nft,
uint256 c_nftId
) {
_seller = payable(msg.sender);
_startingPrice = c_startingPrice;
_startAt = block.timestamp;
_expiresAt = block.timestamp + DURATION;
_discountRate = c_discountRate;
require(
_startingPrice >= _discountRate * DURATION, "starting price < min"
);
_nft = IERC721(c_nft);
_nftId = c_nftId;
}
function price() public view returns (uint256) {
uint256 timeElapsed = block.timestamp - _startAt;
uint256 discount = _discountRate * timeElapsed;
return _startingPrice - discount;
}
function buy() external payable _auctionNotExpired {
uint256 currentPrice = price();
require(msg.value >= currentPrice, "ETH < price");
_nft.transferFrom(_seller, msg.sender, _nftId);
uint256 refund = msg.value - currentPrice;
if (refund > 0) {
payable(msg.sender).transfer(refund);
}
}
modifier _auctionNotExpired() {
require(block.timestamp < _expiresAt, "auction expired");
_;
}
}
Congratulations! You have now implemented a dutch auction smart contract. This type of contract can be used in all sorts of applications and I hope it is a baseline for something awesome you build.